This is a simple example on how to setup a web application which uses OpenJPA on WebSphere v8.0 using a data source provided via jndi. The development environment is Eclipse and Apache Maven. The data source we are using will connect to an Oracle SQL Developer 11g Database using the Oracle Thin Client driver. Code for this can be found at https://github.com/jamie3/openjpa-jndi-websphere.
Pre-Requisites
- Eclipse
- Oracle SQL Developer 11g or equivalent database
- An existing database already setup in Oracle
- WebSphere v8.0
Web Application Project
- Create a new maven project called “openjpa-jndi-websphere" and set its packaging to "war".
- Update the pom by adding the following:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>openjpa-jndi-websphere</groupId> <artifactId>openjpa-jndi-websphere</artifactId> <version>1.0</version> <packaging>war</packaging> <properties> <openjpa.version>2.2.1</openjpa.version> </properties> <dependencies> <dependency> <groupId>org.apache.openjpa</groupId> <artifactId>openjpa</artifactId> <version>${openjpa.version}</version> </dependency> <dependency> <groupId>javax.servlet</groupId> <artifactId>servlet-api</artifactId> <version>2.5</version> <scope>provided</scope> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.apache.openjpa</groupId> <artifactId>openjpa-maven-plugin</artifactId> <version>2.2.2</version> <configuration> <includes>**/model/*.class</includes> <excludes>**/model/XML*.class</excludes> <addDefaultConstructor>true</addDefaultConstructor> <enforcePropertyRestrictions>true</enforcePropertyRestrictions> </configuration> <executions> <execution> <id>enhancer</id> <phase>process-classes</phase> <goals> <goal>enhance</goal> </goals> </execution> </executions> <dependencies> <dependency> <groupId>org.apache.openjpa</groupId> <artifactId>openjpa</artifactId> <!-- set the version to be the same as the level in your runtime --> <version>${openjpa.version}</version> </dependency> </dependencies> </plugin> </plugins> </build> </project>
Note: Open-JPA Version should be set to 2.2.1 or whatever is provided by your IBM WebSphere container.
- In the project properties select "Project Facets".
- Select the WebSphere Web (Co-existence) and (Extended) boxes.
EAR Project
Here we will create the EAR which will allow us to deploy the WAR onto WebSphere.
- Create a new maven project called "openjpa-jndi-websphere-ear" with packaging "ear".
- Update the pom with the following:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>openjpa-jndi-websphere</groupId> <artifactId>openjpa-jndi-websphere-ear</artifactId> <version>1.0</version> <packaging>ear</packaging> <dependencies> <dependency> <groupId>openjpa-jndi-websphere</groupId> <artifactId>openjpa-jndi-websphere</artifactId> <version>1.0</version> <type>war</type> </dependency> </dependencies> <build> <plugins> <plugin> <artifactId>maven-ear-plugin</artifactId> <version>2.10</version> <configuration> <version>6</version> <defaultLibBundleDir>lib</defaultLibBundleDir> <modules> <webModule> <groupId>openjpa-jndi-websphere</groupId> <artifactId>openjpa-jndi-websphere</artifactId> <contextRoot>/openjpa-example</contextRoot> </webModule> </modules> </configuration> </plugin> </plugins> </build> </project>
Setup Data Source in Eclipse
Here we are going to setup the data source in Eclipse which will allow us to connect to the Oracle database and create our JPA Entities later.- In Eclipse open the “Data Source Explorer” Perspective.
- Add a new database connection
- Select Oracle connection profile. I’m using the Oracle SQL Developer 11g
- Select Oracle Thin Driver 11g
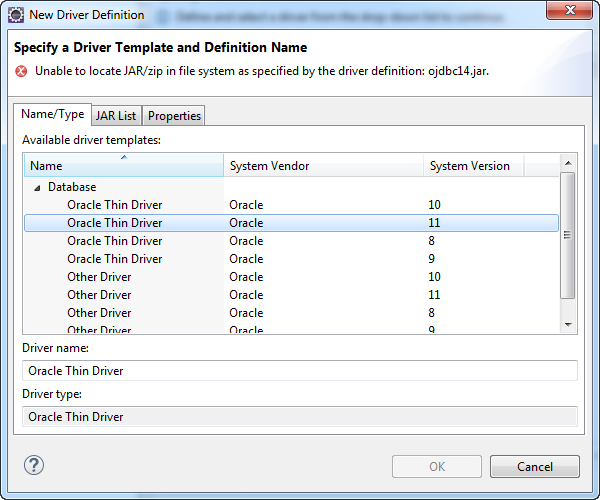
- Click the JAR List tab
- Click Add JAR/Zip button
- Select the jar to use (E.g. C:\oraclexe\app\oracle\product\11.2.0\server\jdbc\lib\ojdbc6_g.jar
- In the Properties section add your connection information.
- Make sure the connection parameters are valid by clicking the "Test Connection" button.
- Right click on the project and select “Configure > Convert to JPA Project”.
- Under JPA Implementation select the Type = Disable Library Configuration. Doing this means you need to use Maven to enhance the JPA entities. If this prompt doesn't appear you can find it by right clicking on the project and selecting "Project Facets".
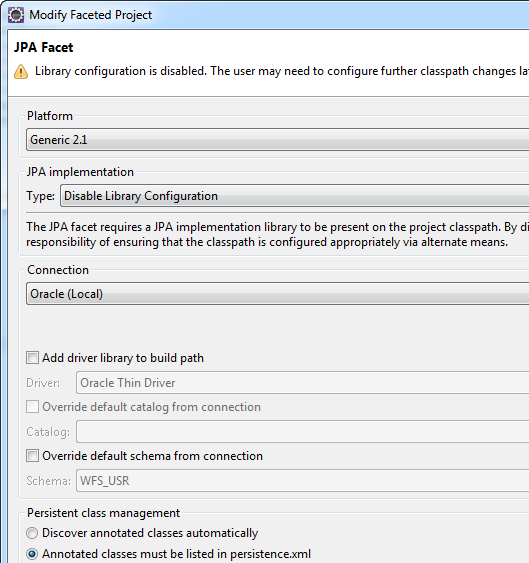
Create JPA Entities
Here we will create our JPA Entity beans using the Eclipse JPA tooling.
- Right click on the project and create a new “JPA Entities from Table”
- On the next screen make sure you select the Oracle connection followed by the schema and tables you want to generate the JPA entity classes. I have a table called sample table.
- In the "Generate Custom Entities" wizard make sure you enter the "source folder" and "package". I usually put my entity classes in a separate package from all my other classes, in this case openjpa.example.model. Also, my database table has a primary key so I've also selected "identity" as the "Key generator".
- In my example a file called SampleTable.java was created and also a persistence.xml.
- Modify the persistence.xml file and add the following
In my example the YOUR_JNDI_NAME is "jdbc/myexample". In your case it will be whatever JNDI Data Source you have previously setup in WebSphere.
- Also set the transaction-type="RESOURCE_LOCAL" in the
.
Create Servlet
Here we will create some Java code such that we can verify JPA is working properly.
- Create a new Java class
package openjpa.example; import java.util.List; import javax.persistence.EntityManager; import javax.persistence.EntityManagerFactory; import javax.persistence.Persistence; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import openjpa.example.model.SampleTable; public class MyServlet extends HttpServlet { private EntityManagerFactory emf; public void init() throws ServletException { super.init(); System.out.println("Initializing servlet"); this.emf = Persistence.createEntityManagerFactory("openjpa-jndi-websphere"); } @Override public void destroy() { if (this.emf != null) { this.emf.close(); this.emf = null; } } @Override protected void doGet(HttpServletRequest request, HttpServletResponse response) { try { EntityManager em = emf.createEntityManager(); System.out.println("Fetching data from database"); List results = em.createNamedQuery("SampleTable.findAll", SampleTable.class).getResultList(); response.getWriter().write("Results: " + results.size()); } catch (Throwable t) { t.printStackTrace(); } } }
web.xml
- Create a new web.xml in the src/main/webapp/WEB-INF folder in your openjpa-jndi-websphere project.
- Update the web.xml with the following:
<servlet> <servlet-name>servlet</servlet-name> <servlet-class>openjpa.example.MyServlet</servlet-class> </servlet> <servlet-mapping> <servlet-name>servlet</servlet-name> <url-pattern>*</url-pattern> </servlet-mapping>
Build
- From eclipse or the command line build the openjpa-jndi-websphere project followed by the openjpa-jndi-websphere-ear project. Basically "mvn install".
Test
Afterwards you can deploy the EAR project onto WebSphere. I've chosen to do this in Eclipse by right clicking on the openjpa-jndi-websphere-ear and selecting "Debug > Debug on Server". This of course requires WebSphere to be setup as a J2EE server in Eclipse.
You can invoke the doGet() method on the MyServlet class by going to the following URL http://localhost:9080/openjpa-example/.
The servlet should query the database and output the results to the web browser.